Script properties and fields
Every script can contain various fields and properties. By default Flax shows all public fields and properties in the Properties window so user may edit them (undo/redo is supported).
Script
using FlaxEngine;
public class MyScript : Script
{
public float Field1 = 11;
public Color Field2 = Color.Yellow;
public DirectionalLight Field3 { get; set; }
}
#pragma once
#include "Engine/Scripting/Script.h"
#include <Engine/Core/Math/Color.h>
#include <Engine/Level/Actors/DirectionalLight.h>
#include <Engine/Scripting/ScriptingObjectReference.h>
API_CLASS() class GAME_API MyScript : public Script
{
API_AUTO_SERIALIZATION();
DECLARE_SCRIPTING_TYPE(MyScript);
API_FIELD() float Field1 = 11;
API_FIELD() Color Field2 = Color::Yellow;
API_FIELD() ScriptingObjectReference<DirectionalLight> Field3;
// [Script]
void OnEnable() override;
void OnDisable() override;
void OnUpdate() override;
};
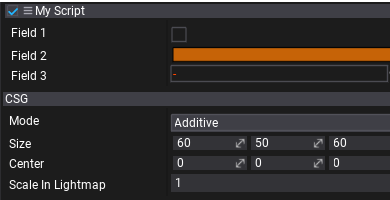
Attributes
If you want to hide a public property or a field simply use HideInEditor attribute.
[HideInEditor]
public float Field1 = 11;
API_FIELD(Attributes="HideInEditor")
float Field1 = 11;
If you want to don't serialize a public property or a field use NoSerialize attribute.
[NoSerialize]
public float Field1 = 11;
API_FIELD(Attributes="NoSerialize")
float Field1 = 11;
To learn more about using attributes see this page.
To learn more about scripts serialization see this page.